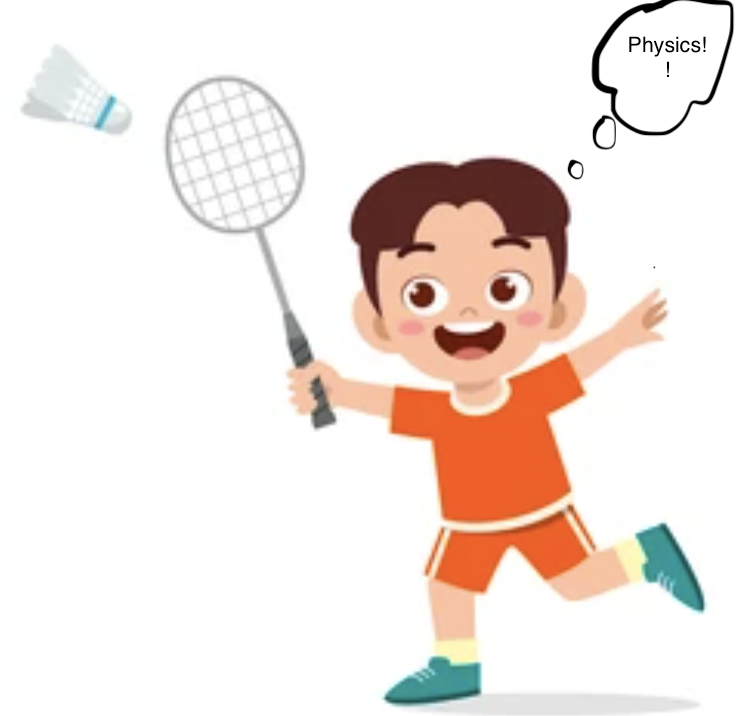
As an experimental physicist, I am always curious on whether I can extract accurate information in a situation where I have an enormous amount of experimental data whereas I left with apparently no direct theoretical/conceptual knowledge on it. The world around us is described with well formulated physics-based models. We can predict the orbit of satellites or future response of nanomaterials to external stimuli given the initial conditions or current knowledge. But this type of physics-based models is not effective enough to address the open questions in physics quickly if we are not equipped with sufficient information to describe it theoretically. For instance, future memory technology based on spintronics requires lot of optimizations of data to understand the factors affecting switching dynamics of magnetic tunnel junctions or to express the physical phenomena affecting spin transfer torque or spin orbit torque efficiency. In such a scenario where there is little information about underlying concepts/phenomena responsible for the extracted data, data driven models based on machine learning (ML) would be a better choice compared to physics based mathematical models. On the contrary, we can use physics-based modeling if we have proper understanding about the system and knows to express it through mathematical equations. ML algorithms are based on learning patterns from experience. This experience is gained through the input we have given to the machine learning model during training stage as data. As data represents underlying physics, it can be restated as machine learning can learn from physics or physics-based models as well. The approach of combining physics or physics-based models with ML is an exciting prospect of ML modeling called hybrid modeling scheme.
How does an ML model learn physics?
Simple! If you have ever played football, volleyball, badminton or any sports of this sort, you would have eventually learned to give the better or perfect shot. This perfect shot depends on many complex variables such as impact on the ball, angle of your hand or foot, air resistance and so on. But without having any awareness of the complex underlying physics, you succeeded in predicting the accurate path of the ball. i.e., you have learned the right movement from experience to predict the accurate path of the ball. As simple as it is, ML algorithms or models also resembles how human brain learn from experience. Once the training stage, which is comparatively complex phase of ML modeling using input data is finished, giving accurate predictions on new data is as simple as that of giving a perfect shot with acquired experience.
Demonstration of ML modeling
The following code snippet trains a machine learning model to classify audio signals into speech or music. From the labeled example audios, the algorithm learns to distinguish between speech and music over time.
# Import libraries
import os
import pandas as pd
from sklearn.model_selection import train_test_split
import tensorflow as tf
from tensorflow.python.keras.layers import Dense
from tensorflow.python.keras.models import Sequential
from tensorflow.python.keras.initializers import RandomUniform
import keras
import matplotlib.pyplot as plt
# Set values for clean data visualization
labelsize = 12
width = 5
height = width / 1.618
plt.rc('font', family ='serif')
plt.rc('text', usetex = True)
plt.rc('xtick', labelsize = labelsize)
plt.rc('ytick', labelsize = labelsize)
plt.rc('axes', labelsize = labelsize)
# Import refined dataSet
datasrc = 'MasterData.csv'
essentialdata = pd.read_csv('Data/'+datasrc, low_memory=False).dropna()
essentialdata = essentialdata.replace({'Speech': 0, 'Music': 1})
essentialdata = essentialdata.sample(frac=1)
features = essentialdata.drop(['Filename', 'Label'], axis=1).values
labels = essentialdata.loc[:,['Label']].values
# Perform Train-Test Split
X_train, X_test, y_train, y_test = train_test_split(features, labels, test_size=0.2, shuffle = True)
# Define the Neural Network Model
def create_model():
model = Sequential()
model.add(Dense(64, activation = 'relu', input_shape= X_train[0].shape))
model.add(Dense(512, kernel_initializer=RandomUniform(minval=-0.05, maxval=0.05),
kernel_regularizer=tf.keras.regularizers.l2(0.001),
activation=tf.nn.relu))
model.add(Dense(128, activation = 'relu',
kernel_regularizer=tf.keras.regularizers.l2(0.001)))
model.add(Dense (128, activation = 'relu',
kernel_regularizer=tf.keras.regularizers.l2(0.001)))
model.add(tf.keras.layers.Dropout(0.5))
model.add(Dense (64, activation = 'relu',
kernel_regularizer=tf.keras.regularizers.l2(0.001)))
model.add(Dense(2, kernel_initializer=RandomUniform(minval=-0.05, maxval=0.05),
kernel_regularizer=tf.keras.regularizers.l2(0.001),
activation=tf.nn.softmax))
model.compile(optimizer=keras.optimizers.RMSprop(learning_rate=0.0001),
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
return model
# Create a basic model instance
model = create_model()
# Give path to the location where trained model is to be saved
checkpoint_path = "./TrainedModel/"
checkpoint_dir = os.path.dirname(checkpoint_path)
# Create a callback that saves the model's weights
cp_callback = tf.keras.callbacks.ModelCheckpoint(filepath=checkpoint_path,
save_weights_only=True,
verbose=1)
# Train the model with callback
history = model.fit(X_train,
y_train,
batch_size = 32,
epochs = 20,
validation_split = 0.20,
callbacks=[cp_callback])
# Load Saved Model
modeltoDeploy = create_model()
# Loads the weights
checkpoint_path = "./TrainedModel/"
checkpoint_dir = os.path.dirname(checkpoint_path)
modeltoDeploy.load_weights(checkpoint_path)
ndatapoints = features.shape[0]
# Re-evaluate the model
loss, acc = modeltoDeploy.evaluate(X_test, y_test, verbose=2)
stats = "Restored model, accuracy: {:5.2f}\% on {} data points".format(100 * acc, ndatapoints)
print(stats)
# Plot the loss graph
fig1, ax = plt.subplots()
fig1.subplots_adjust(left=.16, bottom=.2, right=.99, top=.90)
plt.plot(history.history['loss'])
plt.plot(history.history['val_loss'])
plt.xlabel('Epochs')
plt.ylabel('Loss')
plt.title(stats)
plt.legend(['Training', 'Validation'], loc='upper left')
fig1.set_size_inches(width, height)
plt.savefig('Graphs/Train_Valiation_Loss_'+str(ndatapoints)+'.png', dpi=300)
plt.close()
# Plot the accuracy graph
fig2, ax = plt.subplots()
fig1.subplots_adjust(left=.16, bottom=.2, right=.99, top=.97)
plt.plot(history.history['accuracy'])
plt.plot(history.history['val_accuracy'])
plt.xlabel('Epochs')
plt.ylabel('Accuracy')
plt.title(stats)
plt.legend(['Training', 'Validation'], loc='upper right')
fig2.set_size_inches(width, height)
plt.savefig('Graphs/Train_Valiation_Accuracy_'+str(ndatapoints)+'.png', dpi=300)
plt.close()